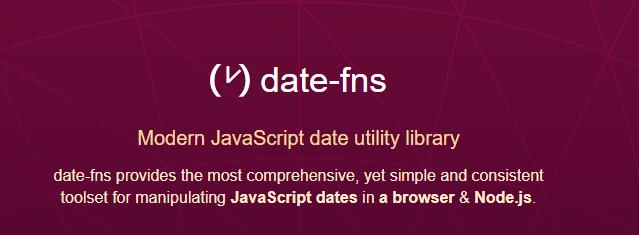
日期工具库date-fns介绍
是一个处理时间的工具库,之前我们用的最多的是moment。date-fns工具库体积小,但是常规处理基本都囊括了。
下载安装
npm install --save date-fns
引入:按需引入
import {xxx} from date-fns
import { zhCN } from "date-fns/locale"; // 设置locale
下面是现目前项目中用到的几个方法:
1.formatRFC3339():转RFC3339格式
参数:
formatRFC3339(date: number | Date, options?: {
fractionDigits?: 0 | 3 | 1 | 2 | undefined;
} | undefined): string // 第二个参数是精度
例子:
console.log(formatRFC3339(new Date())); // 2021-08-10T11:21:53+08:00
console.log(formatRFC3339(new Date(), { fractionDigits: 3 })); // 2021-08-10T11:27:30.543+08:00
2.format():格式化日期
参数:
format(date: number | Date, format: string, options?: {
locale?: Locale | undefined; // locale为中国时间
weekStartsOn?: 0 | 1 | 2 | 3 | 4 | 5 | 6 | undefined;
firstWeekContainsDate?: number | undefined;
useAdditionalWeekYearTokens?: boolean | undefined;
useAdditionalDayOfYearTokens?: boolean | undefined;
} | undefined): string
例子:
console.log(format(new Date(), "yyyy-MM-dd HH:mm:ss", { locale: zhCN })); // 2021-08-10 11:42:31
console.log(format(new Date(), "yyyy-MM-dd HH:mm:ss")); // 2021-08-10 11:38:44
console.log(format(new Date(), "yyyy-MM-dd")); // 2021-08-10
console.log(format(new Date(), "HH:mm:ss")); // 11:38:44
3.addDays():计算某天加减多少天数是哪一天
参数:
addDays(date: number | Date, amount: number): Date
例子:
console.log(addDays(new Date(), 1)); // Wed Aug 11 2021 11:47:07 GMT+0800 (中国标准时间)
console.log(addDays(new Date(), -1)); // Mon Aug 09 2021 11:47:07 GMT+0800 (中国标准时间)
console.log(addDays(new Date(), -29)); // Mon Jul 12 2021 11:47:07 GMT+0800 (中国标准时间)
类似函数还有:
addMonths():几个月后的日期,
addHours():几个小时后的日期,
addMinutes():几分钟后的日期
addWeeks():几个周后的日期
4.getDate(): 获取传入日期的天
参数:
getDate(date: number | Date): number
例子:
console.log(new Date()); // Tue Aug 10 2021 13:56:16 GMT+0800 (中国标准时间)
console.log(getDate(new Date())); // 10
console.log(getDate(addDays(new Date(), -2))); // 8
类似函数还有:
getYear():获取传入时间的年份,
getMonth():获取传入时间的月份,
getDay():获取传入时间是星期几
5.isAfter():判断日期1是否在日期2之后
参数:
isAfter(date: number | Date, dateToCompare: number | Date): boolean
例子:
console.log(isAfter(new Date(), new Date("2020-12-11"))); // true
console.log(isAfter(addDays(new Date(), -2), new Date())); // false
类似函数还有:
isBefore():判断日期1是否在日期2之前
6.isSameDay():判断两个日期是否是同一天(同年同月同日)
参数:
isSameDay(dateLeft: number | Date, dateRight: number | Date): boolea
例子:
console.log(isSameDay(new Date(), new Date("2021-8-10"))); // true
类似函数还有:
isSameMonth():判断两个日期是否在同一月(同年同月)
isSameHour():判断两个时间是否是同一个小时(同一天的小时判断)
7.startOfMonth():获取传入日期所在月份的第一天的时间
参数:
startOfMonth(date: number | Date): Date
例子:
console.log(startOfMonth(new Date("2020-12-11"))); // Tue Dec 01 2020 00:00:00 GMT+0800 (中国标准时间)
类似函数还有:
startOfYear():获取传入日期所在年份的第一天的时间
startOfDay():获取传入日期的零点日期
startOfWeek():获取传入日期所在星期的第一天的零点时间
startOfWeek(date: Date | number, options?: {
locale?: globalThis.Locale | undefined;
weekStartsOn?: 0 | 1 | 2 | 3 | 4 | 5 | 6 | undefined;
} | undefined): Date // 因为中国星期的起始时间和国际的星期开始时间有差异,所以需要设置了可选项
8.isToday():判断传入日期是否是当天
参数:
isToday(date: Date | number): boolean
例子:
console.log(isToday(new Date()); // true
类似函数还有:
isYesterday():判断传入日期是否是昨天
isTomorrow():判断传入日期是否是明天
9.subDays(): 获取传入日期n天以前的日期
参数:
subDays(date: number | Date, amount: number): Date
例子:
console.log(subDays(new Date("2020-12-11"), 20)); // Sat Nov 21 2020 08:00:00 GMT+0800 (中国标准时间)
类似函数还有:
subMonths():获取传入日期之前n个月的时间
subHours(): 获取传入时间之前n小时的时间
subMinutes(): 获取传入时间之前n分钟的时间
10.differenceInDays():计算两个时间之间相差的天数
参数:
differenceInDays(dateLeft: number | Date, dateRight: number | Date): number
例子:
console.log(differenceInDays(new Date("2021-10-20"), new Date("2021-10-1"))); // 19
类似函数还有:
differenceInMonths():计算两个时间之间相差的月份
differenceInYears():计算两个时间之间相差的年数
differenceInHours():计算两个时间之间相差的小时
differenceInWeeks():计算两个时间之间相差的周数
11.parseISO():将字符串形式的日期转换成Date格式的日期
参数:
parseISO(argument: string, options?: {
additionalDigits?: 0 | 1 | 2 | undefined;
} | undefined): Date
例子:
console.log(parseISO("2021-12-19")); // Sun Dec 19 2021 00:00:00 GMT+0800 (中国标准时间)
评论
匿名评论
隐私政策
你无需删除空行,直接评论以获取最佳展示效果